Downloading files using PowerShell can be efficiently achieved through five primary methods. First, the 'Invoke-WebRequest' command enables straightforward downloads with built-in error handling. Second, 'Start-BitsTransfer' utilizes the Background Intelligent Transfer Service for optimized network performance. Third, the 'WebClient' object simplifies file transfers with its easy-to-use interface. Fourth, in PowerShell V7, 'Invoke-RestMethod' facilitates downloads from web APIs, streamlining HTTP interactions. Finally, Task Scheduler allows for the automated execution of download scripts at defined intervals. Each method offers unique advantages for different scenarios, ensuring effective file management in your workflows. Further exploration exposes even more capabilities.
Key Takeaways
- Use 'Invoke-WebRequest' for straightforward file downloads, incorporating error handling for reliable results.
- Utilize 'Start-BitsTransfer' for efficient background downloads, optimizing network bandwidth and providing error handling options.
- Leverage the 'WebClient' object for a simple interface, allowing synchronous and asynchronous file downloads in PowerShell.
- Employ 'Invoke-RestMethod' to easily download files from web APIs, with built-in error handling and file integrity checks.
- Automate downloads using Task Scheduler to run PowerShell scripts at specified intervals, ensuring robust error management.
Using Invoke-WebRequest Command
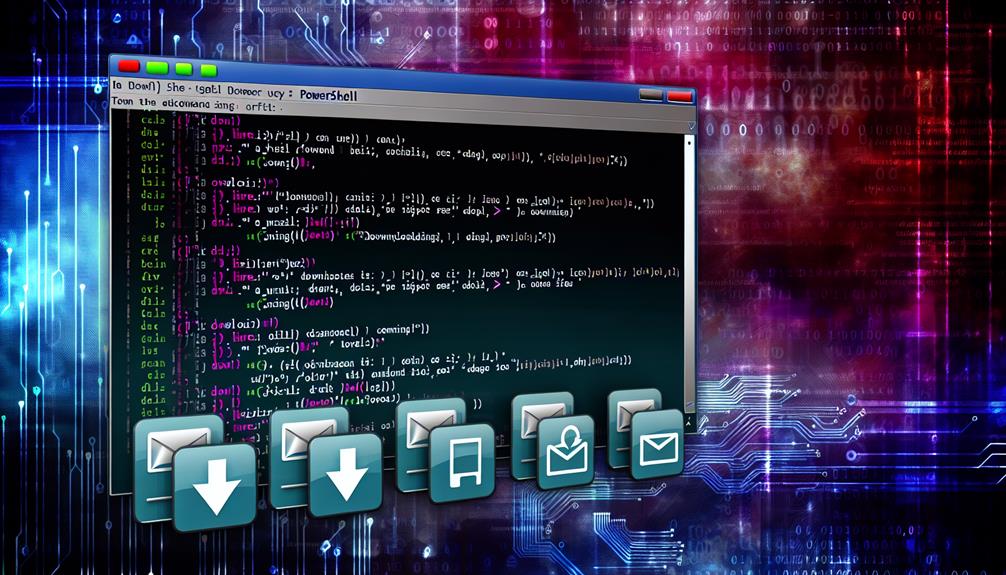
The Invoke-WebRequest command in PowerShell is an invaluable tool for downloading files from the internet. This command offers users a streamlined approach to retrieve web content, making it essential for automation and scripting tasks. With the rise of remote work opportunities, having skills in automation, such as using PowerShell for file management, can greatly enhance your productivity and job prospects in the current job market remote customer service benefits. To initiate a download, the basic syntax involves specifying the URL and the destination path, such as 'Invoke-WebRequest -Uri "http://example.com/file.zip" -OutFile "C:\pathoile.zip"'.
Incorporating error handling is imperative to guarantee robustness in your scripts. Utilize the '-ErrorAction' parameter to manage exceptions, allowing your script to proceed gracefully if the download fails. For example, '-ErrorAction Stop' will halt execution upon encountering an error, enabling you to implement additional recovery procedures.
Furthermore, file validation is essential to confirm that the downloaded content is intact and as expected. After downloading, you can use the 'Test-Path' cmdlet to verify the existence of the file and check its integrity using hash verification methods, such as 'Get-FileHash'. By integrating these practices into your PowerShell workflows, you can enhance reliability and maintain a professional standard in your file management processes.
Utilizing Start-BitsTransfer
For users seeking a more robust method of downloading files in PowerShell, utilizing Start-BitsTransfer presents an efficient alternative to Invoke-WebRequest. This command leverages the Background Intelligent Transfer Service (BITS), allowing file transfers to occur in the background, optimizing network bandwidth usage, and providing the ability to resume failed downloads.
The Start-BitsTransfer command offers various options such as specifying the source and destination paths, controlling the priority of the transfer, and managing transfer states. However, users may encounter Start-BitsTransfer errors, which can stem from issues such as network connectivity or incorrect file paths. Proper handling of these errors enhances the reliability of file downloads.
Here's a quick reference table summarizing key Start-BitsTransfer options and common errors:
Start-BitsTransfer Options | Common Start-BitsTransfer Errors |
---|---|
-Source | 0x80040154: Unspecified error |
-Destination | 0x800C0011: Network connection lost |
-Priority | 0x80070002: File not found |
-Asynchronous | 0x80070057: Invalid parameter |
Downloading With Webclient Object
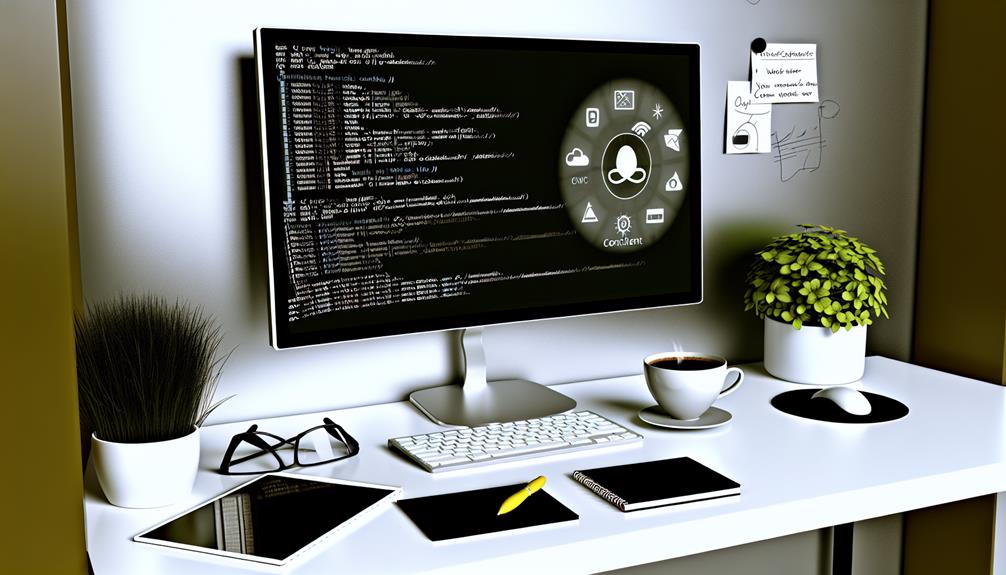
Another method for downloading files in PowerShell is by utilizing the WebClient object. This object provides a straightforward interface for sending data to and receiving data from a resource identified by a URI. Understanding WebClient basics is essential for leveraging its capabilities effectively, just as gaining insight into educational platforms can enhance children's learning experiences.
To begin, instantiate the WebClient object in your PowerShell script. This can be done with the command '$client = New-Object System.Net.WebClient'. Once you have your WebClient object, you can use it to download files with the 'DownloadFile' method. The syntax is simple: '$client.DownloadFile("URL", "DestinationPath")'. Replace "URL" with the direct link to the file you want to download, and "DestinationPath" with the path where you wish to save the file.
WebClient offers various file download options, including asynchronous methods, which can enhance performance in scripts requiring multiple downloads. By utilizing the WebClient object, you can easily manage file transfers while maintaining clean and efficient code. This method is particularly advantageous for users looking to integrate file downloads seamlessly into their PowerShell workflows.
Employing Powershell V7's Invoke-Restmethod
Utilizing PowerShell V7's Invoke-RestMethod provides a powerful way to download files directly from web APIs or other online resources. This cmdlet simplifies the process by handling the HTTP request and response seamlessly. Here's a concise overview of its functionality:
Action | Description | Example Command |
---|---|---|
Basic Download | Download content from a URL | 'Invoke-RestMethod -Uri "http://example.com/file" -OutFile "localfile.txt"' |
Error Handling | Manage errors during the download process | 'try { Invoke-RestMethod -Uri "url" } catch { Write-Error "Download failed" }' |
File Validation | Confirm the file integrity post-download | 'if (Test-Path "localfile.txt") { "File downloaded successfully." }' |
Scheduling Downloads With Task Scheduler
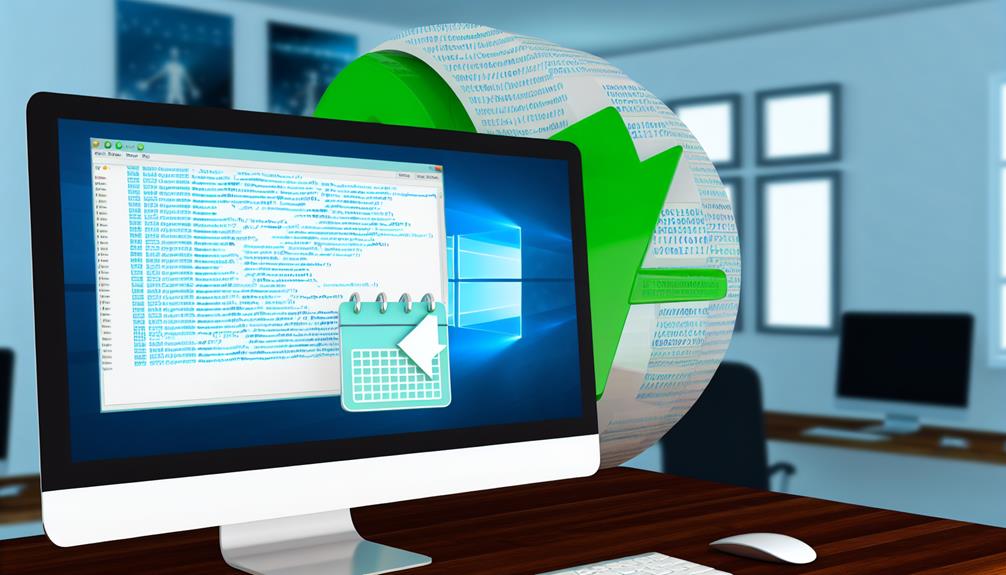
After successfully downloading files using PowerShell V7's Invoke-RestMethod, you may want to automate the process for regular tasks. Scheduling downloads with Task Scheduler allows you to run your PowerShell script at specified intervals, ensuring timely access to needed files without manual intervention. Additionally, understanding market trends can enhance your investment strategy, as discussed in investment opportunities provided by Freedom Trading Co.
To begin, open Task Scheduler and select "Create Basic Task." Name your task and set a trigger based on your needs—daily, weekly, or monthly. In the action step, choose "Start a program" and enter 'powershell.exe' in the Program/script field. In the "Add arguments" section, input the path to your PowerShell script, including any necessary parameters.
It's essential to include robust error handling within your script to manage potential issues during downloads. Utilize try-catch blocks in your PowerShell code to gracefully handle exceptions, ensuring that the task does not fail silently. Additionally, you can log errors to a file for later review.
Frequently Asked Questions
Can I Download Multiple Files Simultaneously Using Powershell?
Yes, you can download multiple files simultaneously using PowerShell by leveraging parallel downloads. This can be achieved through the use of download scripts that implement asynchronous operations. Utilizing cmdlets such as 'Start-Job' or 'Invoke-WebRequest' in conjunction with 'ForEach-Object' allows for concurrent execution, greatly improving download efficiency. By structuring your scripts accordingly, you can optimize bandwidth usage and reduce overall download time, facilitating a more productive workflow.
How Do I Check the Download Progress in Powershell?
To check the download progress in PowerShell, you can utilize the 'Invoke-WebRequest' cmdlet, which provides a progress indication during file downloads. By using the '-OutFile' parameter, you can specify the destination file. Additionally, employing the '-UseBasicPipelines' option allows you to monitor download speed and progress through the console output. This approach not only keeps you informed but also enhances your overall experience while managing file downloads effectively.
Are There Any File Size Limits When Downloading?
When downloading files, it is crucial to take into account potential file size limits imposed by various factors, including the operating system, application constraints, or network configurations. These limits can impact download performance, especially in environments with strict bandwidth management policies. For best results, verify that the file sizes do not exceed the permissible thresholds set by your system or network, facilitating smoother and more efficient downloads tailored to your specific technical requirements.
What File Types Can I Download Using Powershell?
PowerShell supports the downloading of various file formats, including text files (.txt), images (.jpg, .png), documents (.pdf, .docx), and executable files (.exe). The versatility of cmdlet usage, such as 'Invoke-WebRequest' and 'Start-BitsTransfer', allows users to handle these diverse file types efficiently. Familiarity with these cmdlets can enhance productivity, enabling users to automate tasks and manage files seamlessly, thereby fostering a sense of belonging within the technical community.
Can I Resume Interrupted Downloads in Powershell?
Yes, you can resume interrupted downloads in PowerShell by implementing robust download management techniques. Utilizing the '-Resume' parameter with the 'Invoke-WebRequest' command allows for continuation from the last downloaded byte, guaranteeing efficient error handling. This feature is particularly beneficial in unstable network conditions, where interruptions may occur. By incorporating these methods, users can enhance their downloading experience and guarantee file integrity, fostering a sense of community among technical professionals.